Setup Knit UI Component
This page will help you get started with Knit. You'll be up and running in a jiffy!
Once you have signed up on our webapp, you will get API Keys for making Knit API calls. Once your have the API Key, you need to do 3 things for your users to start interacting with Knit's embedded UI Auth Component:
- Setup your Backend to get auth session token.
- Setup your Frontend to use the auth session token and fire up Knit's UI Auth Component.
- After an integration is complete, pass the Integration Details to your Backend. If integration validation is successful, Integration Id along with API Key can be used for making API calls post integration.
Let me take you through these steps in detail.
1. Setup your Backend to get auth session token
In your backend, set up a POST request to initialize a KnitAuth session and get a authSessionToken using Auth Session Creation API
See the snippet below.
// replace the following fields in body with your values
var body = {
originOrgId: "", // Your end user's organization org id
originOrgName: "", // Your end user's organization org name
originUserEmail: "", // Your end user's organization user email
originUserName: "", // Your end user's organization user name
};
// Replace <API_KEY> with your API_KEY
var API_KEY = "<API_KEY>";
var headers = {
Authorization: "Bearer " + API_KEY,
};
fetch(`https://api.getknit.dev/v1.0/auth.createSession`, {
method: "POST",
body: JSON.stringify(body),
headers: headers,
})
.then((res) => {
res.json();
console.log(res.msg.token);
})
.catch((error) => {
console.error(error);
});
import okhttp3.*;
import org.json.JSONObject;
import java.io.IOException;
public class AuthCreateSession {
public static void main(String[] args) throws IOException {
String originOrgId = ""; // Your end user's organization org id
String originOrgName = ""; // Your end user's organization org name
String originUserEmail = ""; // Your end user's organization user email
String originUserName = ""; // Your end user's organization user name
String requestUrl = "https://api.getknit.dev/v1.0/auth.createSession";
// Replace <API_KEY> with your API_KEY;
String API_KEY = "<API_KEY>"
// Create the request body
JSONObject requestBody = new JSONObject();
requestBody.put("originOrgId", originOrgId);
requestBody.put("originOrgName", originOrgName);
requestBody.put("originUserEmail", originUserEmail);
requestBody.put("originUserName", originUserName);
try {
// Create the HTTP client and request
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, requestBody.toString());
Request request = new Request.Builder()
.url(requestUrl)
.method("POST", body)
.addHeader("Authorization", "Bearer " + API_KEY)
.addHeader("Content-Type", "application/json")
.build();
// Send the request and handle the response
Response response = client.newCall(request).execute();
JSONObject jsonResponse = new JSONObject(response.body().string());
String token = jsonResponse.getJSONObject("msg").getString("token");
// get the token
System.out.println("Auth Session Token : " + token);
} catch(Exception e) {
System.out.println(e);
}
}
}
import requests
import json
# Fill in the following fields with your values
originOrgId = "" # Your end user's organization org id
originOrgName = "" # Your end user's organization org name
originUserEmail = "" # Your end user's organization user email
originUserName = "" # Your end user's organization user name
API_KEY = "<API_KEY>" # Replace <API_KEY> with your API_KEY
headers = {
"Authorization": "Bearer " + API_KEY
}
url = "https://api.getknit.dev/v1.0/auth.createSession"
body = {
"originOrgId": originOrgId,
"originOrgName": originOrgName,
"originUserEmail": originUserEmail,
"originUserName": originUserName
}
response = requests.post(url, headers=headers, json=body)
if response.status_code == 200:
response_data = json.loads(response.content)
print(response_data['msg']['token'])
else:
print("Error:", response.status_code, response.content)
An auth session token is a short lived token and helps us in securely identifying your end user's details while doing an integration. This will eventually help your team in communicating any issues that might have happened if an integration goes awry.
API Key is a sensitive token and should never be passed to the Frontend.
That's why you need to generate an auth session token on your Backend and pass that to your Frontend.
Once you have an auth session token, pass that to your frontend to fire up the Knit UI component.
2. Setup your Frontend to use the auth session token and fire up Knit's UI Auth Component
Knit UI component will help you build native integration experiences for your end users. The component is entirely hosted as a single JS file in here . In this section, we will see how to use this JS file in different web frameworks for building native integration experiences.
Knit UI Component with React.js
Please click here for more details.
Knit UI Component with Vanilla Javascript
Please click here for more details.
Knit UI Component with Angular.js
Please click here for more details.
Knit UI Component with VueJS
Please click here for more details.
If all goes will you should see Knit's UI Component fire up.
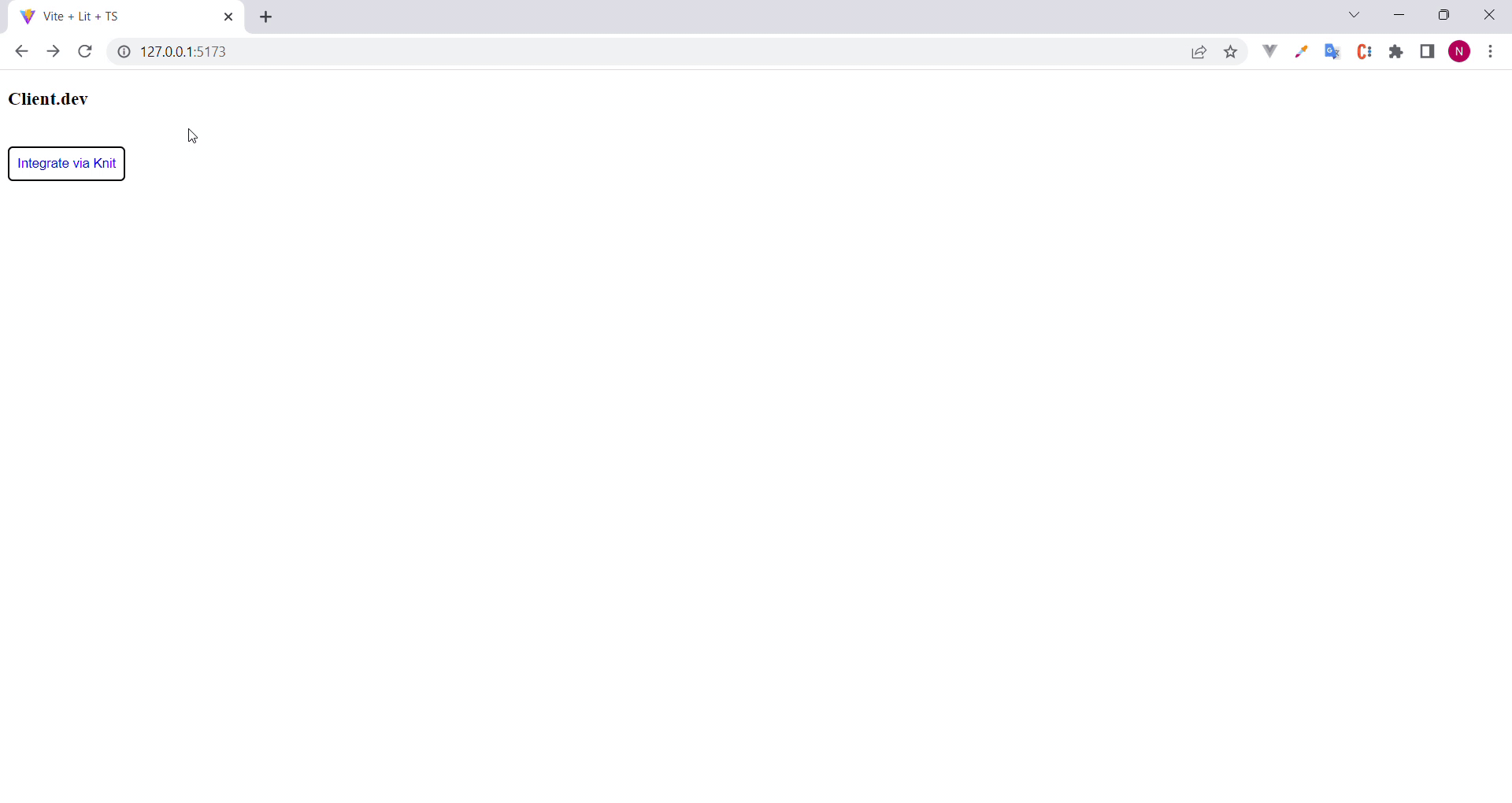
3. After an integration is complete, pass the Integration Details
to your Backend
Integration Details
to your BackendAt Knit, we make sure that every integration is good to go before you make API calls. Once your user enters and submits their credentials in the UI Auth Component, we’ll run some checks to validate the credentials and return the integration validation data to the frontend. Your users can also see the errors and resolutions on the UI Auth component enabling them to self fix these issues without involving your team.
You should pass the integrationDetails
to your backend and store it securely in a persistent store. You'll need the integrationId
to make API calls to Knit.
What's an Integration Id?
Whenever your user integrates with an app, you'll get an
integrationId
for that integration.This uniquely identifies the user + app combination and is how we can differentiate between integrated accounts. This id would be needed when making API calls, and it would also be present in the webhooks we send to you.
There are two kinds of responses that you expect when the user submits their credentials:
-
Success: Client credentials are good to go. Sample payload below:
{ "integrationDetails": { "integrationId": "b29fVEtxc1U0TjNvaWN4Z3h1dUxXaDc5bTpodWJzcG90", "appId": "hubspot", "categoryId": "CRM", "originOrgId": "org1", "success": true } }
-
Error: There were some errors reported. For each error we will also share a descriptive message. Also these errors will be listed as issues on our web app under
Issues
. Sample payload below:{ "integrationDetails": { "integrationId": "b29fVEtxc1U0TjNvaWN4Z3h1dUxXaDc5bTpodWJzcG90", "appId": "hubspot", "categoryId": "CRM", "originOrgId": "org1", "success": false, "errors": [ { "code": "E002", "description": "Invalid API Key - api key provided isn't valid", "resolution": "Please make sure you have API access and then ask admin to fill the correct credentials" } ] } }
You can start making API calls only after the
success
flag is true in this payload
Updated 2 days ago